感知机
题目要求如下:

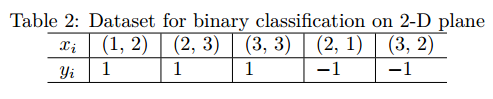
首先我们产生数据(注意,我们的data后面都加上一个1,是为了计算感知机里的偏置也就是常数项):
1 | import numpy as np |
感知机计算函数如下:
1 | def sign(w,x): |
然后就是计算感知机系数的过程,如果一轮迭代后权重发生改变,则继续循环,否则说明权重已经收敛,就停止计算,返回权重
1 | def calulate_w(iters): |
最后就是将我们计算得到的结果画出来,如下所示:
1 | def main(): |
[-7 9 -1]
结果图和w计算结果如上图所示。
SVM分类(使用sklearn)
题目要求如下:

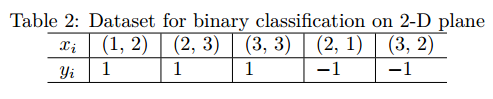
产生数据同上:
1 | from numpy import * |
计算svm的系数,调包即可:
1 | def compute_svm(x,y): |
最后得出结果,画图如下:
1 | def main(): |
('weight for svm:', array([-1., 2.]))
可得图和权重结果。